この記事は、Processing 3.5.4 の Java言語におけるコールバック処理の実装を備忘録として書いています。
現在制作中のblanClass_Shouko ABEさんとのコラボレーションプロジェクト”KesuNeco(仮)”というアートアプリ!? のようなのを製作中、その際に使用したコールバック処理を備忘録として簡単に解説。
コールバック処理とは【初心者向け】
非同期での処理が完了した後に結果が実行されるような時に使える。例えば、クラスAの処理とクラスBの処理が連携して、処理を分離させたり、任意のタイミングでイベントを発動させるための仕掛け。
今回のコールバック処理の特徴
呼び出された非同期状態の処理を完了したら、コールバックが返ってくる仕組みです。
- クラスAにコールバックを実装
- クラスAの中でクラスBを経由してコールバックに登録
- クラスAが呼び出され、Bの処理を完了してから再びAの処理に戻ってくる。
参考にさせて頂いたSOFTBASE JPさんページです。
Referenced Link : https://qiita.com/softbase/items/94984fa7821ecbc02f5b
サンプルコードでのコールバックの使用例
制作中の”KesuNeco(仮)”の中では、ユーザーが書いた落書きをネコが消してゆくのだが、ネコが消した瞬間にコールバックが欲しかったのだ。なのでこのサンプルコードはその仕組だけを抽出して、視覚的にわかりやすくイメージ化し、コーンソール上でも実行経過の文字が出るようにした。
このサンプルコードについて、
- Processingのmainのdraw()関数(*1)の中では、Runした後に出てくる画面上でマウスが押された時にclass Aのオブジェクトがインスタンス化され呼び出されます。
- class Aの中でBのオブジェクトをインスタンス化しています。
その処理と同時にBを経由してclass Aのオブジェクトをコールバックに登録しておきます。 - class Aの処理を実行中に条件を満たすとBの処理が開始します。B処理が終了すると、はじめに登録しておいたclass Aの処理が再び呼び出されます。
(*1) Processing(Java)では最初に実行される関数がmain(String[] args)ではなく、setup()とdraw()のため、コールバックのインターフェースはclass Aにimplements しました。(ちょっと冗長なコードの書き方になってしまいのが課題、もっと簡単なやり方で書ける方法あれば、ぜひコメントください!)
サンプルコードの実行経過の解説
コールバックをイメージ化視覚的にわかりやすく作ったつもりなので解説いたす、、、。
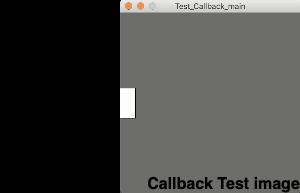
- サンプルコードをRunすると画面の左端中央に小さな白い四角がでてきます。画面上でマウスをクリックすると四角が右に動き出します。
- 条件 : 四角が画面右端に触れるとコールバックの仕掛けが呼び出されます。
- コールバックが呼び出されると、まずBの処理が始まります。
- Bの処理 : まず2秒間止まります、そしてその後、四角が大きくなり左側に向かって動き出します。
- 四角が左端のスタート位置に到達すると、再び四角が右に動き出し一連のシーケンスが繰り返されます。
- 四角のサイズが画面サイズぎりぎりになるまでこの動作は続きます。
以下、サンプルコード【開発環境 : Processing 3.5.4】
// Test_Callback_main.pde
// Development environment by Processing 3.5.4
/*
Demonstration of this sample code.
Run the sample source code.
There is a small white rectangle(RECT) on the center of left side.
First, Click on display
Second, RECT is moving to Right, and when RECT is touch on the frame of display,
CALLBACK starts to run. At that first step of CALLBACK is just stop 2 seconds.
Then RECT is getting bigger than before and move back to Left side.
Last, RECT reaches at the first start point.
And repeat this sequence until RECT size is almost the same size of display height.
* 2020 @author sharagu <https://sharagublog.post-past.com>
*/
float x = 10;
float h = 50;
boolean started = false;
boolean backed = false;
color r = color(200, 0, 0);
color w = color(240);
A a;
void setup() {
size(300, 300);
background(120);
rectMode(CENTER);
}
void draw() {
rect(x, 150, 30, h);
//RECT is moving to Right
if(started) {
x += 5;
stroke(200,0,0);
backed = false;
if(x >= width-20) a.colorChange(r);
if(x >= width) a.callB();
}
//RECT is moving to LEFT
if(backed) {
x -= 5;
if(x <= 0) started = true;
}
//Stop
if(h >= height) {
noLoop();
println("end");
return;
}
}
//instantiate Object class_A
void mousePressed() {
started = true;
a = new A();
}
// interface_CallBack.pde
interface Callback {
void call();
}
// class_A.pde
class A implements Callback {
B callMan;
A() {
//instantiate Object class_B and register class_A to class_B
callMan = new B();
callMan.register(this);
}
public void callB() {
//calling AnyTask of class_B
println("calling...");
callMan.doAnyTask();
}
public void colorChange(color red) {
fill(red);
}
//interface_CallBack function "call()"
@Override
public void call() {
// calling BACK from class_B
println("CALLBACK!!!!!!!!" + "\n");
started = false;
backed = true;
}
}
// class_B.pde
class B {
private Callback notify;
B() {}
// register class_A to interface_CallBack
void register(Callback callback) {
notify = callback;
}
void doAnyTask() {
/* calling from class_A
Do any Task of B....*/
try {
println("wait for 2 seconds" + "\n" + "...");
// 2秒間処理を止める
Thread.sleep(2000);
} catch (InterruptedException e) {
}
h += 30;
stroke(0, 0, 200);
fill(w);
/*Done the Task of B....
calling BACK to class_A*/
notify.call();
}
}
【English】Description of this sample code.
Sample code : Callback function by Processing_Java
Development environment by Processing 3.5.4
- Run the sample source code.
- There is a small white rectangle(RECT) on the center of left side.
- First, Click on display
- Second, RECT is moving to Right, and when RECT is touch on the frame of display,
- CALLBACK starts to run. At that first step of CALLBACK is just stop 2 seconds. Then RECT is getting bigger than before and move back to Left side.
- Last, RECT reaches at the first start point. And repeat this sequence until RECT size is almost the same size of display height.
コメント